Rotation Fix Attempt 1 - Demo 08¶
Purpose¶
Fix the rotation problem from the previous demo in a seemingly intuitive way, but do it inelegantly.
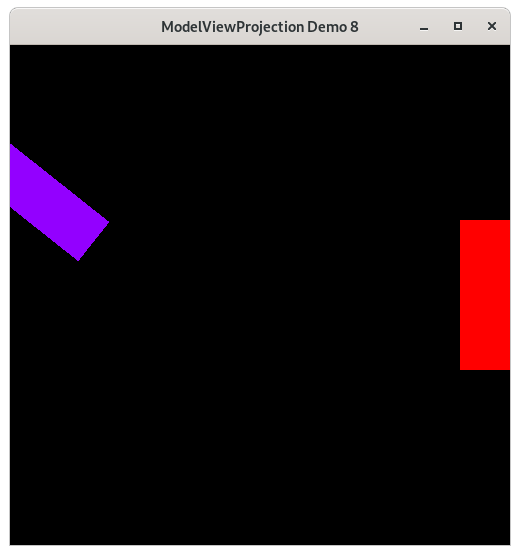
Demo 08¶
How to Execute¶
Load src/demo08/demo.py in Spyder and hit the play button
Move the Paddles using the Keyboard¶
Keyboard Input |
Action |
---|---|
w |
Move Left Paddle Up |
s |
Move Left Paddle Down |
k |
Move Right Paddle Down |
i |
Move Right Paddle Up |
d |
Increase Left Paddle’s Rotation |
a |
Decrease Left Paddle’s Rotation |
l |
Increase Right Paddle’s Rotation |
j |
Decrease Right Paddle’s Rotation |
Description¶
The problem in the last demo is that all rotations happen relative to World Space’s (0,0) and axes. By translating our paddles to their position before rotating, they are rotated around World Space’s origin, instead of being rotated around their modelspace’s center.
In this demo, we try to solve the problem by making a method to rotate around a given point in world space, in this case, the paddle’s center.
109class Vertex:
145 def rotate_around(self: Vertex, angle_in_radians: float, center: Vertex) -> Vertex:
146 translate_to_center: Vertex = self.translate(-center)
147 rotated_around_origin: Vertex = translate_to_center.rotate(angle_in_radians)
148 back_to_position: Vertex = rotated_around_origin.translate(center)
149 return back_to_position
150
Within the event loop, this seems quite reasonable
218while not glfw.window_should_close(window):
238 glColor3f(paddle1.r, paddle1.g, paddle1.b)
239
240 glBegin(GL_QUADS)
241 rotatePoint: Vertex = paddle1.position
242 for paddle1_vertex_ms in paddle1.vertices:
243 paddle1_vertex_ws: Vertex = paddle1_vertex_ms.translate(paddle1.position)
244 paddle1_vertex_ws: Vertex = paddle1_vertex_ws.rotate_around(
245 paddle1.rotation, rotatePoint
246 )
247 paddle1_vertex_ndc: Vertex = paddle1_vertex_ws.uniform_scale(scalar=1.0 / 10.0)
248 glVertex2f(paddle1_vertex_ndc.x, paddle1_vertex_ndc.y)
253 # draw paddle 2
254 glColor3f(paddle2.r, paddle2.g, paddle2.b)
255
256 glBegin(GL_QUADS)
257 rotatePoint: Vertex = paddle2.position
258 for paddle2_vertex_ms in paddle2.vertices:
259 paddle2_vertex_ws: Vertex = paddle2_vertex_ms.translate(paddle2.position)
260 paddle2_vertex_ws: Vertex = paddle2_vertex_ws.rotate_around(
261 paddle2.rotation, rotatePoint
262 )
263 paddle2_vertex_ndc: Vertex = paddle2_vertex_ws.uniform_scale(scalar=1.0 / 10.0)
264 glVertex2f(paddle2_vertex_ndc.x, paddle2_vertex_ndc.y)
265 glEnd()
All we did was add a rotate around method, and call it, with the paddle’s center as the rotate point.
Although this works for now and looks like decent code, this is extremely sloppy, and not thought out well at all. We apply a transformation from paddle space to world space, then do the inverse, then rotate, and then do the first transformation from paddle space to world space again.
The images of the transformation sequence below should show how brain-dead it is, and the Cayley graph is gross.
But from this we will learn something important.
translating back to the origin
resetting the coordinate system
rotating
resetting the coordinate system
and them translating them back to the paddle space origin
Cayley Graph¶
Note, this is gross, and the edge from the paddlespace to itself doesn’t even make any sense, but the author did not know how else to represent this code.
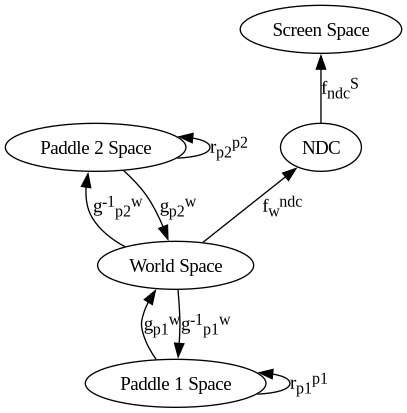