Rotate the Square - Demo 12¶
Purpose¶
Rotate the square around its origin.
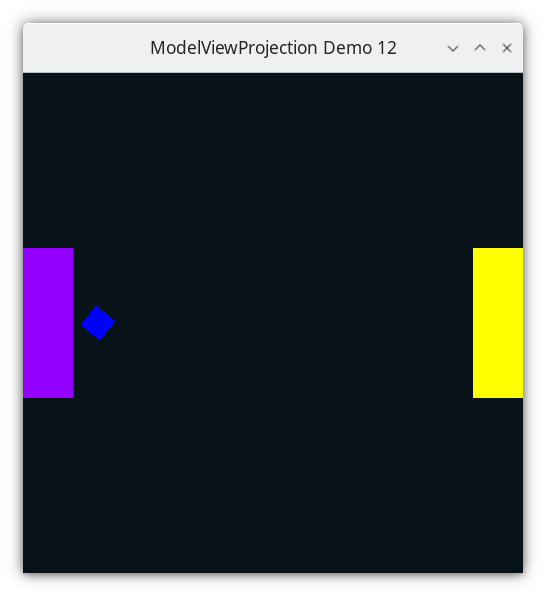
Demo 12¶
How to Execute¶
Load src/demo12/demo.py in Spyder and hit the play button
Move the Paddles using the Keyboard¶
Keyboard Input |
Action |
---|---|
w |
Move Left Paddle Up |
s |
Move Left Paddle Down |
k |
Move Right Paddle Down |
i |
Move Right Paddle Up |
d |
Increase Left Paddle’s Rotation |
a |
Decrease Left Paddle’s Rotation |
l |
Increase Right Paddle’s Rotation |
j |
Decrease Right Paddle’s Rotation |
UP |
Move the camera up, moving the objects down |
DOWN |
Move the camera down, moving the objects up |
LEFT |
Move the camera left, moving the objects right |
RIGHT |
Move the camera right, moving the objects left |
q |
Rotate the square around its center |
Description¶
Cayley Graph¶
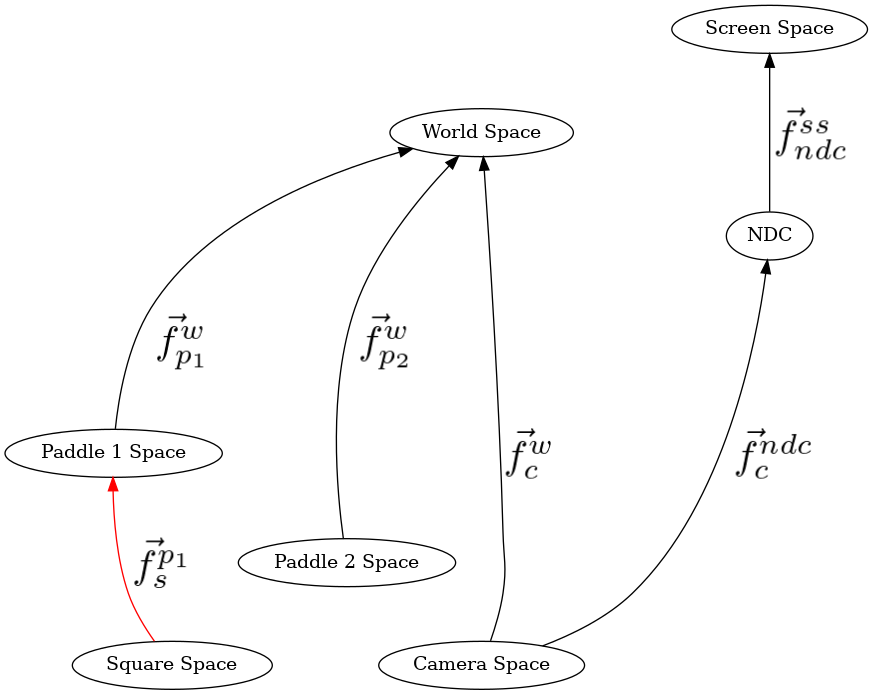
Demo 12¶
Code¶
Make a variable to determine the angle that the square will be rotated.
196square_rotation: float = 0.0
When ‘q’ is pressed, increase the angle.
201def handle_inputs() -> None:
202 global square_rotation
203 if glfw.get_key(window, glfw.KEY_Q) == glfw.PRESS:
204 square_rotation += 0.1
...
Event Loop¶
In the previous chapter, this was the rendering code for the square.
269 glColor3f(0.0, 0.0, 1.0)
270 glBegin(GL_QUADS)
271 for model_space in square:
272 paddle1space: Vertex = model_space.translate(Vertex(x=2.0, y=0.0))
273 world_space: Vertex = paddle1space.rotate(paddle1.rotation).translate(
274 paddle1.position
275 )
276 camera_space: Vertex = world_space.translate(-camera.position_ws)
277 ndc: Vertex = camera_space.uniform_scale(scalar=1.0 / 10.0)
278 glVertex2f(ndc.x, ndc.y)
279 glEnd()
Since we just want to add one rotation at the end of the sequence of transformations from paddle 1 space to square space, just add a rotate call at the top.
272 glColor3f(0.0, 0.0, 1.0)
273 glBegin(GL_QUADS)
274 for square_vertex_ms in square:
275 paddle_1_space: Vertex = square_vertex_ms.rotate(square_rotation).translate(
276 Vertex(x=2.0, y=0.0)
277 )
278 world_space: Vertex = paddle_1_space.rotate(paddle1.rotation).translate(
279 paddle1.position
280 )
281 camera_space: Vertex = world_space.translate(-camera.position_ws)
282 ndc: Vertex = camera_space.uniform_scale(scalar=1.0 / 10.0)
283 glVertex2f(ndc.x, ndc.y)
284 glEnd()
The author is getting really tired of having to look at all the different transformation functions repeatedly defined for each object being drawn.