Introduction¶
Learn how to program in 3D computer graphics in Python!
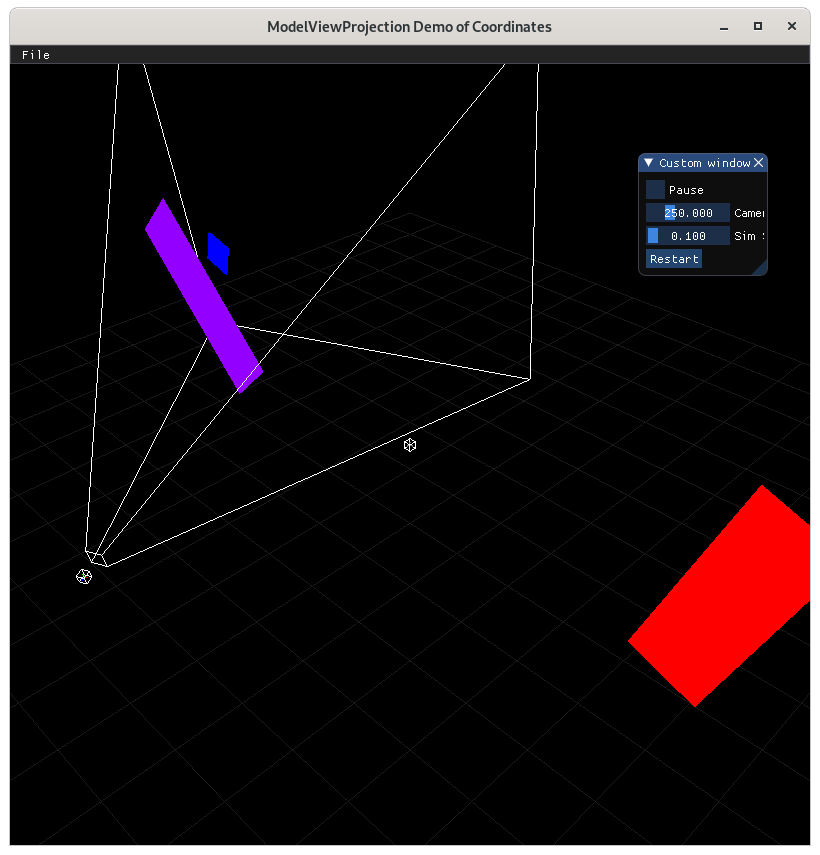
Source code¶
This book references source code, which is at https://github.com/billsix/modelviewprojection
Approach¶
This book takes a “mistake-driven development” approach: instead of handing you polished math formulae, it walks you through building complex graphics applications step by step. Along the way, we’ll make mistakes—and then fix them—so you can see how solutions emerge in real-world programming.
You’ll learn how to place objects in space, draw them relative to others, add a camera that moves over time based on user input, and transform all those objects into the 2D pixel coordinates of your screen. By the end, you’ll understand the foundations of creating first-person and third-person applications or games. The goal? To empower you to build the graphics programs you want, using math you mostly already know.
This book keeps things intentionally simple. The applications we create won’t be particularly pretty or realistic-looking. For more advanced topics, you’ll want to dive into references like the OpenGL “Red Book ” and “Blue Book,” or explore some of the tutorials listed at the end.
While this book fills a huge gap by focusing on the basics in a hands -on way, those other books are fantastic references for diving into advanced topics.
Pre-requisities¶
Basic programming concepts in Python.
YouTube videos
Books
High school trigonometry
Linear Algebra (optional)
3Blue1Brown - Linear Transformations
3Blue1Brown - Matrix Multiplication as Composition
Required Software¶
You will need to install Python. https://realpython.com/installing-python/
Before running this code, you need a virtual environment, with dependencies installed. https://docs.python.org/3/tutorial/venv.html
Windows¶
On Windows, if you use the Developer command prompt, to set up the environment run
python -m venv venv
cd venv\Scripts
activate.bat
cd ..\..\
python -m pip install --upgrade pip setuptools
python -m pip install -r requirements.txt
To run the Spyder IDE to execute the code in the book, open Spyder on the developer command prompt
cd venv\Scripts
activate.bat
cd ..\..\
spyder
MacOS or Linux¶
On MacOS or Linux, on a terminal, to set up the environment, run
python3 -m venv venv
source venv/bin/activate
python3 -m pip install --upgrade pip setuptools
python3 -m pip install -r requirements.txt
To run the Spyder IDE to execute the code in the book, open Spyder on the developer command prompt
source venv/bin/activate
spyder
Linux¶
Install Python3, glfw via a package manager. Use pip and virtualenv to install dependencies
Mac¶
Python Python3 (via anaconda, homebrew, macports, whatever), and use pip and virtualenv to install dependencies.